How to implement a Lottie animation
To implement a Lottie animation, you need to use a Lottie player, which is a library that enables the rendering of Lottie JSON files on various platforms. Lottie players are available for web development, iOS, Android, React Native, and more. Below are basic steps to implement a Lottie animation using the Lottie player for the web:
Lottie Web (JavaScript/HTML):
1. Include Lottie Library:
- Include the Lottie library in your HTML file. You can use a CDN or download the library and host it yourself.
- Note that this is AirBNB’s official player. There are other Lottie players for web out there as well, which can be used:
<script src="https://cdnjs.cloudflare.com/ajax/libs/bodymovin/5.7.6/lottie.min.js"></script>
2. Create HTML Container:
- Add a container element to your HTML where you want the animation to appear.
- Note that this is also AirBNB specific code
<div id="lottie-container"></div>
3. Initialize Lottie Animation:
- Use JavaScript to initialize Lottie with the JSON file and link it to the container.
- Note that this is also AirBNB specific code
<script>
const animationPath = 'path/to/your-animation.json';
const container = document.getElementById('lottie-container');
const animation = lottie.loadAnimation({
container: container,
renderer: 'svg', // or 'canvas'
loop: true,
autoplay: true,
path: animationPath,
});
</script>
);</script>
Replace 'path/to/your-animation.json' with the actual path to your Lottie JSON file.
4. Test Your Website:
- Open your HTML file in a web browser to see the Lottie animation on your website.
Lottie for Other Platforms:
- Lottie iOS (Swift):
- Install Lottie using CocoaPods or Swift Package Manager.
- Load the Lottie animation using AnimationView in your Swift code.
- Lottie Android (Java/Kotlin):
- Add the Lottie library dependency to your Android project.
- Use LottieAnimationView in your XML layout file or programmatically in Java/Kotlin.
- React Native:
- Install the lottie-react-native package.
- Use the LottieView component in your React Native code.
Lottie Files:
When creating Lottie files, you can use Adobe After Effects with the Bodymovin plugin to export animations as JSON files. These JSON files contain the data necessary to render vector animations.
Source code - https://codepen.io/TeamFlash/pen/vYENayx
Bodymovin Library - https://cdnjs.com/libraries/bodymovin
More articles:
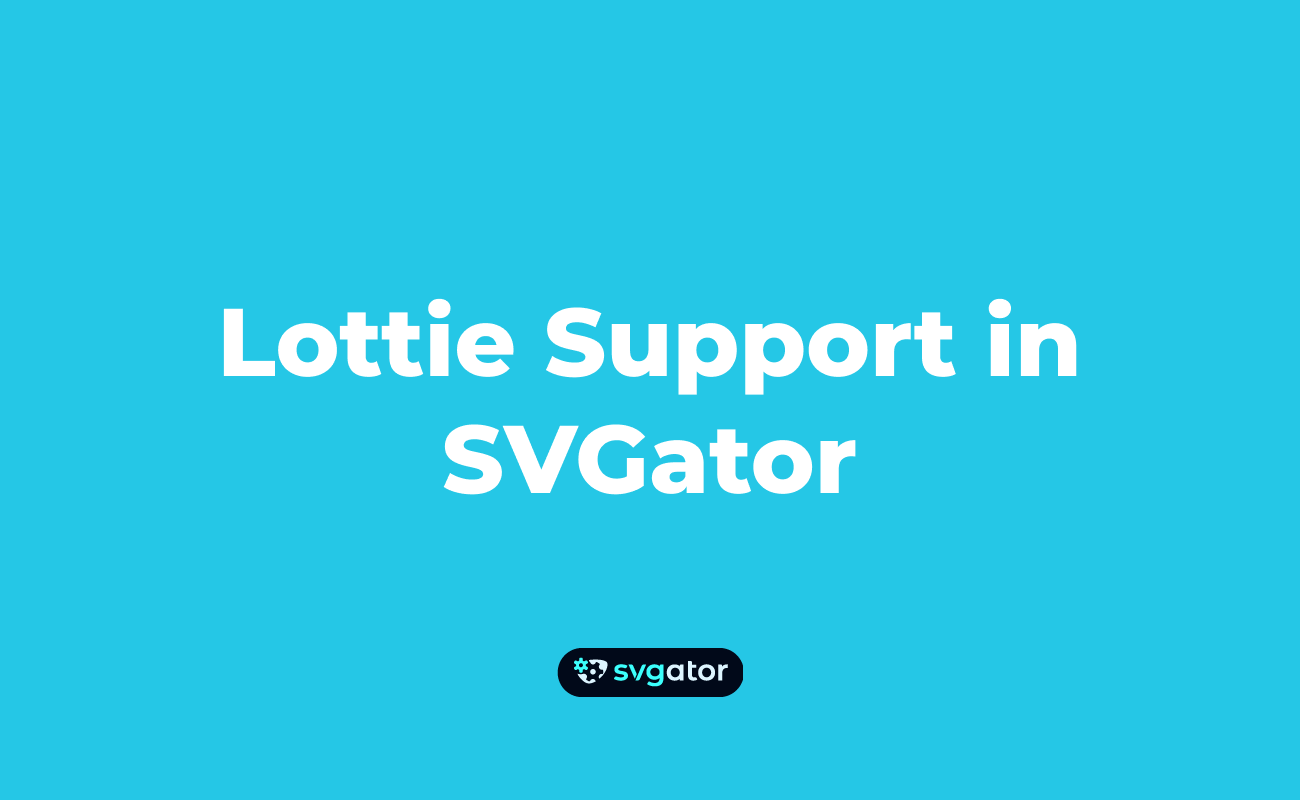
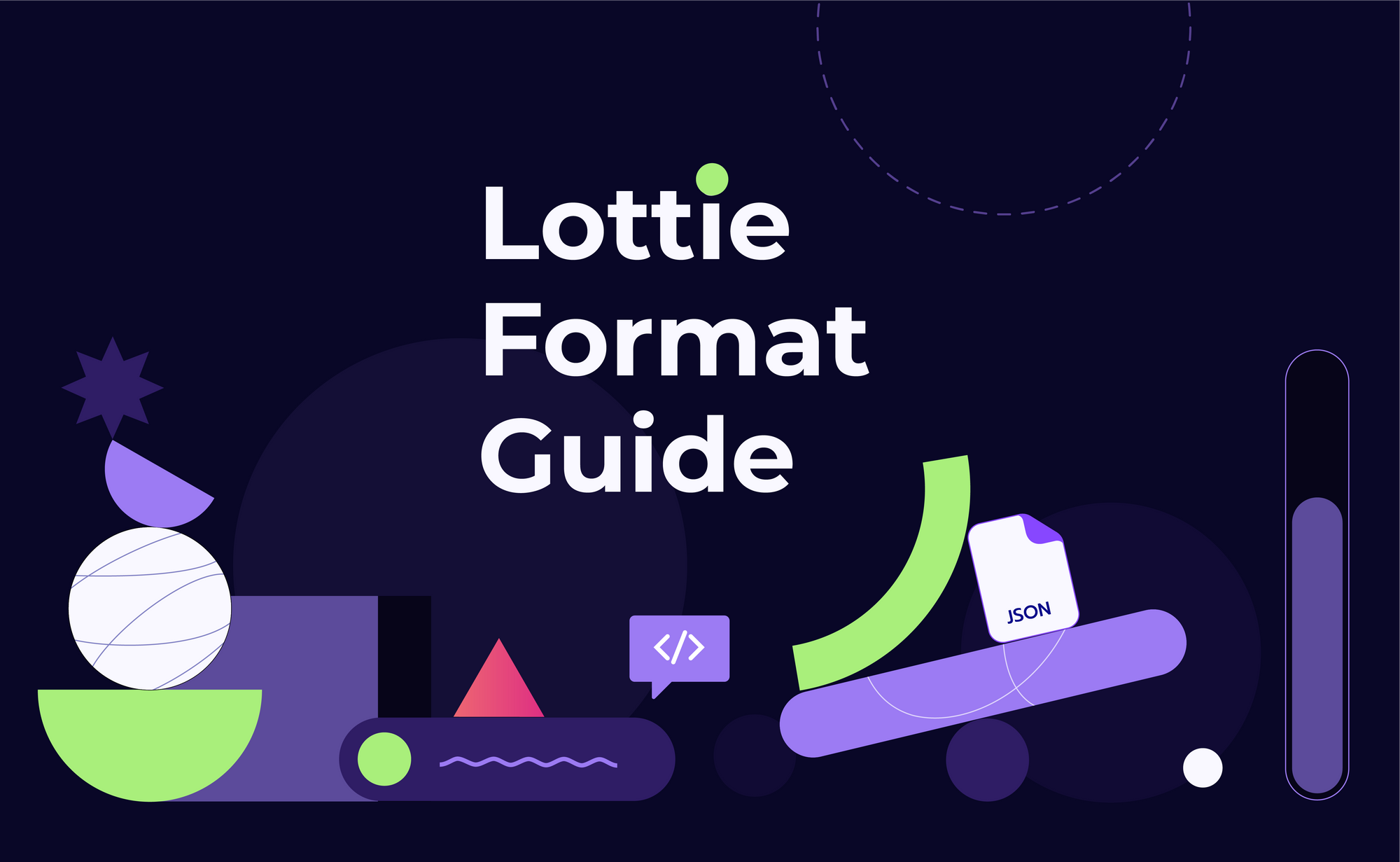
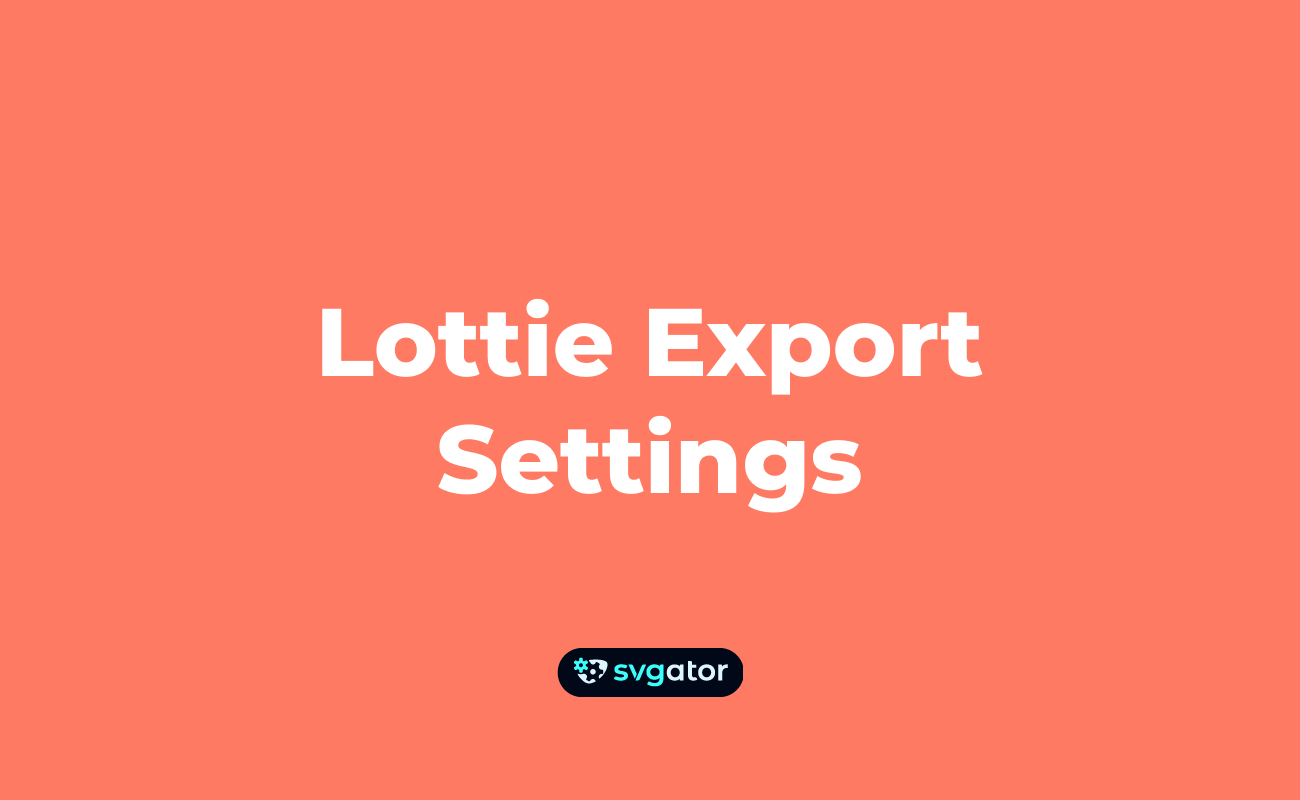
Still got questions? Send us an email to contact@svgator.com and we will get back to you as soon as we can.